Chapter 1
Introducing WPF
Objectives:
Understand the motivation behind WPF
Examine the various ‘flavors’ of WPF applications
Overview the services provided by WPF
Examine the core WPF assemblies and namespaces
Work with the Window and Application class types
Learn the syntax of XAML
Understand the XAML / code relationship
Copyright © Intertech, Inc 2014 Rev: 2
1-1 Introducing WPF
Chapter Overview
Every few years or so, Microsoft introduces a new GUI toolkit. Since the release of .NET 3.0, the latest desktop GUI toolkit is Windows Presentation Foundation (WPF).
In this introductory chapter, you will understand the motivation behind WPF, learn the syntax of XAML, examine the core programming model, and survey several WPF services. Also, you will overview the WPF support provided by Visual Studio and be introduced to the role of Expression Blend.
As you would expect, this chapter provides a foundation for the remainder of the class.
Copyright © Intertech, Inc 2014 Rev: 2
1-2 Introducing WPF
The Motivation Behind WPF
Historically speaking, building a desktop UI under the Windows OS involved using a handful of key services:
•
•
User32: Provided the code required to create windows, controls, and application infrastructure.
•
•
GDI: Provided a framework for rendering 2D graphical data.
DirectX: If an application required high-performance graphical rendering
(multimedia applications, video games, rich interactive front ends, and so on),
DirectX was the way to do so.
Although Windows programmers could make use of these services using raw
C/C++, many UI toolkits were introduced over the years:
•
•
•
•
•
VB6, MFC, Windows Forms, GDI+, and so on.
Each one of them was simply a wrapper around User32 / GDI.
These toolkits still viewed DirectX as an ‘external’ service.
Windows Presentation Foundation (WPF) is a managed GUI toolkit that shipped beginning with .NET 3.0.
•
•
•
The core WPF object model is similar, but not identical to, Windows Forms.
The release of WPF does not imply that Windows Forms is obsolete.
In fact, the .NET platform will support this API for years to come.
Copyright © Intertech, Inc 2014 Rev: 2
1-3 Introducing WPF
Before the release of WPF, UI developers were forced to master a number of related, but ultimately independent, APIs.
•
•
This required developers to ‘switch gears’ when moving from one task (e.g., building a main window) to another (e.g., 3D graphical rendering).
•
•
The end result was a very asymmetrical way to program.
Consider the GUI development world before .NET 3.0:
Desired Functionality Pre-.NET 3.0 Solution
Forms, dialog boxes, controls
Windows Forms, VB6, MFC, and so on
2D graphics System.Drawing.dll (e.g., GDI+) or raw C-based GDI
3D graphics DirectX
Streaming video Windows Media Player API or third party APIs
Fixed / Flow documents Third party APIs
With the release of WPF, things have improved considerably.
•
•
You now have a single symmetrical manner in which to interact with the necessary
GUI infrastructure.
•
Consider the GUI development world as of .NET 3.0 and higher:
Desired Functionality .NET 3.0 and Higher Solution
Forms, dialog boxes, controls Windows Presentation Foundation
2D graphics Windows Presentation Foundation
3D graphics Windows Presentation Foundation
Streaming video Windows Presentation Foundation
Fixed / Flow documents Windows Presentation Foundation
Copyright © Intertech, Inc 2014 Rev: 2
1-4 Introducing WPF
Beyond offering a unified programming model, WPF also provides a clear separation of concerns.
•
•
•
It is possible to separate the look and feel of a GUI application from the programming logic that drives it.
•
•
This is achieved using an XML-based grammar termed XAML (‘zam-el’).
While most WPF applications will make use of XAML, doing so is entirely optional.
This separation of concerns (via XAML) makes it much simpler for graphical designers to build very rich, professional UIs.
•
•
•
Graphically minded individuals can use dedicated design tools such as Microsoft
Expression Blend to generate the XAML.
These XAML files can then be passed to the programming team to add logic to drive the UI (event handlers, method overrides, and so on).
As you will see in the class, a Microsoft Blend project uses exactly the same format as a Visual Studio project.
Beyond the introduction of XAML, WPF also provides a good number of integrated services, including (but not limited to) the following:
•
•
A number of layout managers that provide full control over placement and repositioning of content.
•
•
A built-in style engine, which allows you to define ‘themes’ for a WPF application.
Native use of vector graphics, which allows an image to be automatically resized to fit the size and resolution of the screen hosting the application.
•
•
•
A rich typography API such as support for XPS (XML Paper Specification) documents, fixed documents (WYSIWYG), flow documents, and document annotations (e.g., a Sticky Notes API).
Integrated 2D and 3D rendering services / animation services, which leverage
DirectX for hardware acceleration. In fact, all rendering, even the rendering of UI elements (buttons, and so on) is preformed via DirectX.
Interoperability with previous UI frameworks. For example, a WPF program can use Windows Forms controls or ActiveX controls. As well, a Windows Forms app can use WPF controls.
•
•
Support for audio and video media.
Support for touch screen development (as of .NET 4.0).
Copyright © Intertech, Inc 2014 Rev: 2
1-5 Introducing WPF
Overall, WPF can be considered a ‘supercharged’ UI framework.
•
•
WPF is extremely useful when you need to build highly interactive, stylized front ends.
•
Real-time rotation of 3D bar charts, spinning a portion of a UI to a 45-degree angle, and dynamic shadowing on a rendered image are all possible.
Consider the following WPF application (an example project from Expression
Blend):
•
•
Clicking on any of the stylized buttons on the bottom of the application will rotate and animate a 3D motorcycle prototype.
•
Each button supports a custom animation, performed when the cursor travels over the surface.
•
•
Notice the drop shadows, angled text blocks, etc.
While all of this could be done without WPF, doing so would require a considerable amount of complex code. Here, a majority of the UI is driven by XAML.
Copyright © Intertech, Inc 2014 Rev: 2
1-6 Introducing WPF
Because WPF applications are so graphically intensive, a production-level WPF application may require the aid of a professional graphic designer.
•
•
•
•
You may agree that most .NET programmers are not the best of artists.
Likewise, most artists are not the best of .NET programmers.
Using XAML and tools such as Visual Studio and Expression Blend, each part of the team can use dedicated tools, thus increasing efficiency in development.
•
Remember that the same Visual Studio WPF application can be opened in
Expression Blend (and vice versa).
This is not to suggest that you cannot use WPF to build ‘traditional’ GUIs (gray push buttons, a grid of data, a menu system, and so on).
•
•
•
•
You can certainly use WPF to build traditional business applications.
However, this API is strongly geared for next-generation GUI applications.
WPF is closer to the ‘Hollywood vision’ of what computer applications should look like (e.g., 3D spinning e-mail messages, glowing animated blocks of text, and the like).
Even if you are interested in building a typical LOB application, WPF can still provide a number of key benefits.
•
•
The WPF ‘content’ model and ‘template’ model make it very easy to customize controls.
A LOB application can add a bit of “eye candy” (animations and visual effects) with just a few lines of XAML.
Copyright © Intertech, Inc 2014 Rev: 2
1-7 Introducing WPF
The Flavors of WPF Applications
Like Windows Forms, WPF can be used to build traditional desktop applications.
•
•
Some possibilities include media viewers, thick client front ends to interact with remote data sources, and word processors.
•
At minimum, this flavor of WPF application will make use of the Window and Application types, in addition to various UI elements (status bars, menu systems, dialogs, and so on).
•
You will learn about these types in this chapter.
Like other desktop applications, WPF desktop apps can be deployed via a standard setup program or ClickOnce deployment.
•
•
•
Of course, the target machine must have the .NET 3.0 or higher runtime installed to support WPF applications.
WPF desktop apps can make use of a page-based system, which allows an application to adopt a web-like navigational structure.
•
•
•
Page navigation apps maintain ‘Next’ and ‘Previous’ buttons, a history list, and the ability to share data between pages.
This type of WPF app makes use of various Page objects hosted within a NavigationWindow.
If you are interested in this flavor of WPF program, consult Appendix C.
Copyright © Intertech, Inc 2014 Rev: 2
1-8 Introducing WPF
WPF can also be used to build a new variety of ‘smart clients’ where the app is embedded into a hosting browser.
•
•
•
•
This flavor of WPF is termed an XBAP (XAML Browser Application).
Like navigationally based apps, XBAPs consist of a number of Page objects.
They are deployed from a remote web server (like a ClickOnce app) and integrate into the navigational structure of the host.
•
XBAPs may be hosted within Internet Explorer or Firefox.
This course will not formally cover XBAP construction, however beware that everything you learn about WPF applies directly to XBAP.
•
•
•
The fundamental difference is how an XBAP is deployed.
Do be aware that Visual Studio does provide a WPF Browser Application project template which you may wish to explore.
•
Also, look up the topic WPF XAML Browser Applications Overview in the .NET
Framework SDK for more information if you are interested.
Copyright © Intertech, Inc 2014 Rev: 2
1-9 Introducing WPF
The WPF and Silverlight Relationship
Silverlight is a web-centric subset of .NET / WPF / XAML functionality.
•
•
•
•
Silverlight makes it possible to build highly interactive web plug-ins. Consider
Silverlight to be Microsoft’s answer to Adobe Flash.
Silverlight allows developers to use C# or Visual Basic to build object-oriented plugins.
Silverlight applications can run on multiple operating systems (currently Windows and Mac OS X and various Linux distributions via the open source Moonlight project).
•
Silverlight can be hosted by multiple browsers (IE, Safari, Opera, and Firefox).
This course does not directly cover Silverlight.
•
•
•
The good news is that Silverlight apps are essentially trimmed down WPF apps.
Thus, if you understand the WPF programming model, you will have no problem building Silverlight programs.
•
Consult for more details.
Copyright © Intertech, Inc 2014 Rev: 2
1-10 Introducing WPF
The WPF Documentation System
During the remainder of this class, you will be exposed to a good number of WPF technologies and application types.
•
•
•
This will provide you with a solid foundation on which to build.
Consult the .NET Framework SDK documentation for additional information.
Simply open the ‘Windows Presentation Foundation’ section of the .NET
Framework documentation.
•
•
•
•
You will find many sample projects, white papers, and code examples within the supplied WPF documentation.
Also, the Controls section documents the full functionality of each WPF control
(properties, methods, events).
Do yourself a favor and be sure to consult the help system during this course, and once you are using WPF back in the workplace.
Copyright © Intertech, Inc 2014 Rev: 2
1-11 Introducing WPF
Core WPF Assemblies and Namespaces
Like any .NET technology, WPF is represented by several assemblies.
•
•
•
•
The Visual Studio WPF project templates automatically set references to the core assemblies.
However, if you build WPF apps using other IDEs or via msbuild.exe, you will need to set assembly references manually.
The following key libraries are managed .NET assemblies.
WPF Assembly Meaning in Life
WindowsBase.dll Defines the base infrastructure of WPF, including dependency properties support.
While this assembly contains types used within the WPF framework, the majority of these types can be used within other
.NET applications.
PresentationCore.dll This assembly defines numerous types that constitute the foundation of the WPF GUI layer.
PresentationFramework.dll This assembly—the ‘meatiest’ of the three—defines the WPF controls types, animation and multimedia support, data binding support, and other WPF services.
For all practical purposes, this is the assembly you will spend most of your time working with directly.
System.Xaml.dll This library (which is new to .NET 4.0) provides types to process and manipulate XAML at runtime.
Copyright © Intertech, Inc 2014 Rev: 2
1-12 Introducing WPF
Although these assemblies provide hundreds of types within numerous namespaces, consider this partial list of WPF namespaces:
•
•
•
You will encounter other namespaces during the remainder of this class.
Again, consult the .NET Framework SDK documentation for full details.
WPF Namespace Meaning in Life
System.Windows Here you will find core types such as Application and Window that are required by any WPF desktop project.
System.Windows.Controls Here you will find all of the expected WPF widgets, including types to build menu systems, tool tips, and numerous layout managers.
System.Windows.Markup This namespace defines a number of types that allow XAML markup and the equivalent binary format, BAML, to be parsed.
System.Windows.Media Within these namespaces you will find types to work with animations, 3D rendering, text rendering, and other multimedia primitives.
System.Windows.Navigation This namespace provides types to account for the navigation logic employed by XAML browser applications / desktop navigation apps.
System.Windows.Shapes This namespace defines basic geometric shapes (Rectangle,
Polygon, etc.) used by various aspects of the WPF framework.
Copyright © Intertech, Inc 2014 Rev: 2
1-13 Introducing WPF
The Role of the Application Class
Regardless of which flavor of WPF application you create, they will all make use of the System.Windows.Application type.
•
•
•
•
This class represents a global running instance of a WPF application.
It encapsulates a number of core services such as handling messages, trapping unhandled exceptions, defining common application data, and more.
Unlike Windows Forms, the WPF Application class exposes most services as instance-level members.
•
•
•
Therefore, you will need to create a class extending Application.
This class is commonly termed the application object.
A WPF application will only have a single Application object.
Copyright © Intertech, Inc 2014 Rev: 2
1-14 Introducing WPF
The Application class defines a number of key services.
Here is a partial list of important members:
•
•
Application Property Meaning in Life
Current This static property provides access to the global WPF application object.
This allows any window (or page) to access the application, which is very helpful in that the app object tends to define core functionality for all owned objects (resources, and so on).
MainWindow Allows you to get or set the main window of the WPF application.
StartupUri Typically used within a XAML description to define the resource containing the definition of the main window.
Properties Allows you to define application-wide variables using name / value pairs.
Similar in concept to a ‘session variable’ in a web app in that any part of your WPF program has access to this data.
Windows Provides access to each Window owned by the current WPF application which makes it simple to iterate over open windows to change their states.
Exit
Startup These are two key events that almost all WPF application objects will handle.
DispatcherUnhandledException This event fires when a WPF throws an unhandled exception. This is your last chance to handle the error before the user is presented with a Windows error dialog box.
Copyright © Intertech, Inc 2014 Rev: 2
1-15 Introducing WPF
The application object will define the Main() method of your program, which must be marked with the [STAThread] attribute.
•
•
•
•
This attribute ensures that any legacy COM objects, ActiveX controls, and the WPF controls load into a thread-safe environment.
If you do not mark your Main() method with the [STAThread] attribute, you will receive a runtime exception.
Here is a simple WPF application object:
// C# class MyApp : Application
{
[STAThread] static void Main()
{
// Create an instance of MyApp.
// Handle events, run the application,
// launch the main window, etc.
}
}
' VB
Class MyApp
Inherits Application
STAThread _
Shared Sub Main()
' Create an instance of MyApp.
' Handle events, run the application,
' launch the main window, etc.
End Sub
End Class
Your Main() method will be the place where you typically want to handle the Startup, Exit, and DispatcherUnhandledException events.
•
•
•
•
Also, your application object can define any number of application-wide data points.
You will build a more complete application object later in this chapter.
For now, examine the role of the Window class.
Copyright © Intertech, Inc 2014 Rev: 2
1-16 Introducing WPF
The Role of the Window Type
In addition to the Application type, most desktop WPF applications will make use of the Window type.
•
•
•
This represents the main window of your app, as well as any custom dialog boxes.
As you can see, Window gains a good deal of functionality from its inheritance chain.
You will come to know the role of many of these base classes during the remainder of this class.
•
•
•
Here is a high-level overview of the role of each class in the Window’s inheritance chain.
The ContentControl parent class allows derived types to host a single piece of ‘content’.
•
•
•
WPF content can be composed of any sort of UI elements.
Most WPF controls also have ContentControl in their inheritance chain.
Also, the Window and Page types extend ContentControl.
Copyright © Intertech, Inc 2014 Rev: 2
1-17 Introducing WPF
The WPF content model allows you to radically change the composition of a control with minimal fuss.
•
•
•
•
By way of an example, a Button could maintain an inner StackPanel as ‘content’.
The StackPanel contains an Ellipse and Label.
You will examine the content model in a later chapter. For now, here is a simple example in XAML.
!-- A Button containing a StackPanel as content. --
Button Height = "150" Width = "120"
StackPanel
Ellipse Fill = "Orange" Height = "75" Width = "75"/
Label Content = "OK!" FontSize = "20"
HorizontalAlignment = "Center" /
/StackPanel
/Button
The Control parent class defines a number of members that give derived types
(including Window) their core look and feel.
•
•
Properties exist to establish the control’s opacity, tab order logic, background color, font settings, and so forth.
•
The Control type also provides the infrastructure to apply templates and styles to a UI widget.
FrameworkElement is another key parent class to many UI widgets in that it provides members to control size, tooltips, mouse cursor, and other settings.
•
•
•
This class also provides support for WPF animation and data binding services.
UIElement provides the greatest amount of functionality:
•
•
Events to process mouse and input focus.
Properties to control focus, visibility, and geometric transformation.
Copyright © Intertech, Inc 2014 Rev: 2
1-18 Introducing WPF
Visual provides derived types the core infrastructure to render their UI output.
•
•
•
•
•
Visual provides hit-testing support and coordinates transformation.
Visual is also the connection between WPF and the DirectX subsystem.
Any type extending Visual can be rendered on a Window.
DependencyObject is the parent that provides derived types the ability to work with the WPF ‘dependency property’ model.
•
•
As you will see later, a dependency property makes it possible for a property to receive input from multiple locations.
This is key part of WPF’s template, animation, and data binding services.
Finally, DispatcherObject provides access to the WPF app’s lower-level event queue via the Dispatcher property.
•
•
WPF makes use of a single-thread affinity model, hence the use of [STAThread] in your Main() method.
Introducing WPF
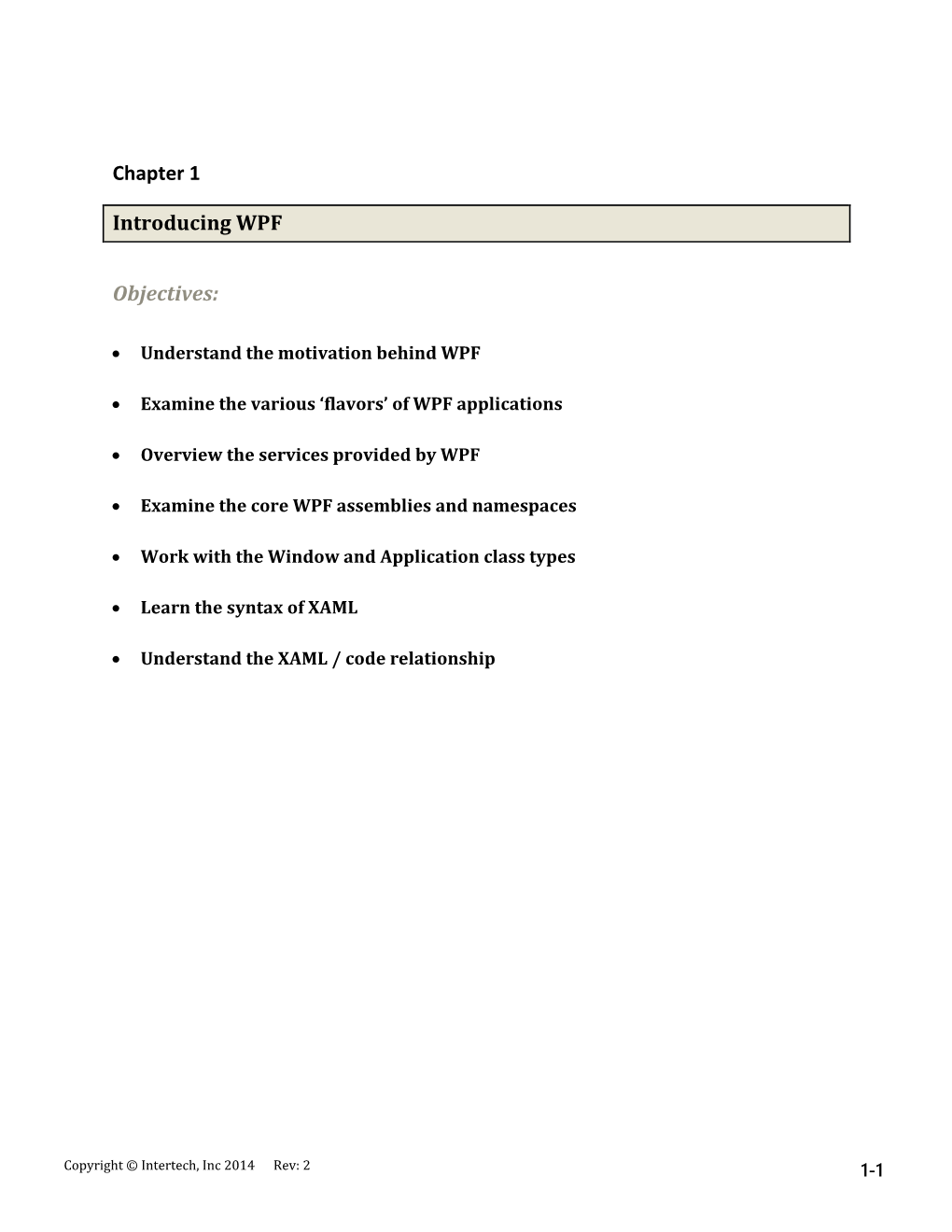