How to change text color in the console
Console.
Rainbows, in all times and ages, have been seen as beautiful. In a console program, colors (like in a rainbow) can be used. These may be less beautiful—but perhaps more useful.
On a console,
text and background colors can be changed. This sometimes makes programs easier to use. Errors and alerts are more noticeable.
Example.
Console has many static methods and properties on it. To see them, type "Console" and press the period. IntelliSense will show you the possible methods.
Here: We see BackgroundColor and ForegroundColor. These are properties, so we do not call them like methods.
Also: We use ResetColor(), which is a method. Is resets all colors on the Console.
Static / C# program that uses BackgroundColor and ForegroundColor using System; class Program { static void Main() { // // 1. Type "Console" and press "." // 2. Select "BackgroundColor". // 3. Press space and "=", then press tab. // Console.BackgroundColor = ConsoleColor.Blue; Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("White on blue."); Console.WriteLine("Another line."); // <-- This line is still white on blue. Console.ResetColor(); } }
Notes, above program.
The System namespace contains the Console class. In Main, the BackgroundColor property is set to ConsoleColor.Blue. ForegroundColor is set to ConsoleColor.White.Property
Note: The two lines, when they are printed, will both have blue backgrounds and white foregrounds.
Also: We see the ResetColor method on Console being used. This sets the colors in your Console back to their defaults.
Example 2.
Writing an entire row of color in the Console may be helpful. It may make a good separator (like a line). Here we refactor the Console code into a separate method.
Tip: In that method, you can change the colors, pad the string, and reset the console.
PadRight: For PadRight the parameter is the Console.WindowWidth minus one. This returns a string that will fill up the Console's width.
Console.WriteLine
Also: If you do not subtract one with the PadRight method, it sometimes incorrectly wraps lines. / C# program that uses padding and colors using System; class Program { static void Main() { // // Write one green line. // WriteFullLine("This line is green."); Console.WriteLine(); // // Write another green line. // WriteFullLine("This line is also green."); Console.WriteLine(); } static void WriteFullLine(string value) { // // This method writes an entire line to the console with the string. // Console.BackgroundColor = ConsoleColor.Green; Console.ForegroundColor = ConsoleColor.DarkGreen; Console.WriteLine(value.PadRight(Console.WindowWidth - 1)); // <-- see note // // Reset the color. // Console.ResetColor(); } }
Example 3.
Here we see a program that displays all the backgrounds and foregrounds possible with the Console. It would be interesting to nest the loops and run though all combinations.
Tip: Microsoft shows a similar program with more complexity. Always use all high-quality references that are available.
Console.BackgroundColor: Microsoft / C# program that shows all console colors using System; class Program { static void Main() { // // This program demonstrates all colors and backgrounds. // Type type = typeof(ConsoleColor); Console.ForegroundColor = ConsoleColor.White; foreach (var name in Enum.GetNames(type)) { Console.BackgroundColor = (ConsoleColor)Enum.Parse(type, name); Console.WriteLine(name); } Console.BackgroundColor = ConsoleColor.Black; foreach (var name in Enum.GetNames(type)) { Console.ForegroundColor = (ConsoleColor)Enum.Parse(type, name); Console.WriteLine(name); } } } Output Black DarkBlue DarkGreen DarkCyan DarkRed DarkMagenta DarkYellow Gray DarkGray Blue Green Cyan Red Magenta Yellow White Black DarkBlue DarkGreen DarkCyan DarkRed DarkMagenta DarkYellow Gray DarkGray Blue Green Cyan Red Magenta Yellow White
Notes, above program.
The program will output a list of ConsoleColor values. If you choose white on blue, you may evoke memories of the BSOD (Blue Screen of Death).
Padding.
Colors are not always the best formatting option. Try focusing on the structure of your output, with padding. We can make columns of text.PadRight, PadLeft
A summary.
We used Console colors, including BackgroundColor and ForegroundColor. The ResetColors method, and the Console.WindowWidth property were also used.
Home
Dot Net Perls / © 2007-2019 Sam Allen. All rights reserved. Written by Sam Allen, email.
How to Change Text Color in the Console
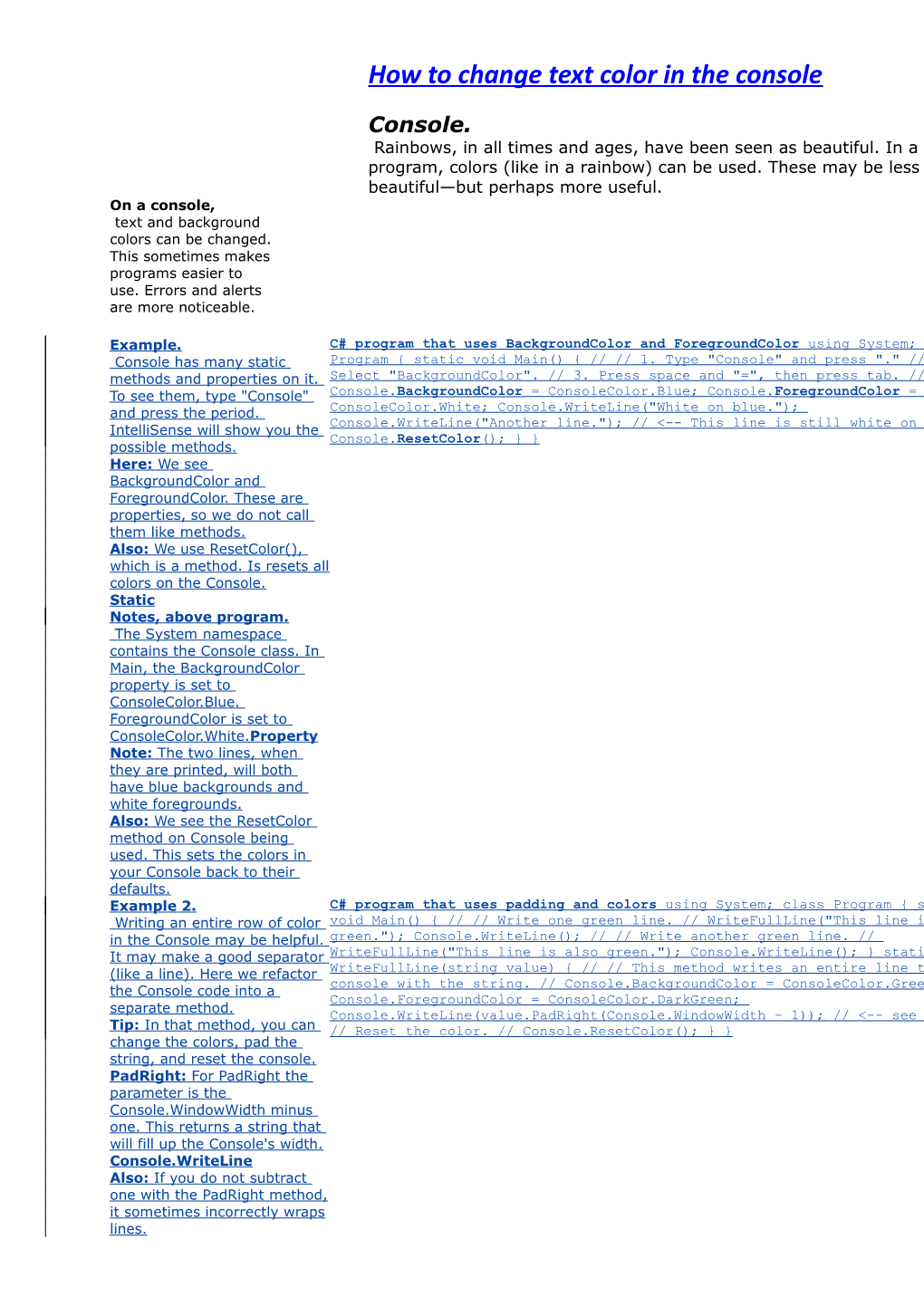